From c22f72dd90f9effcd61243cf28c1d9945b507ef1 Mon Sep 17 00:00:00 2001 From: Akif9748 <akif9748@gmail.com> Date: Sun, 3 Apr 2022 22:03:47 +0300 Subject: [PATCH] Added thread to API --- APIDOCS.md | 17 ++++---- README.md | 10 +++-- routes/api/ApiResponse.js | 7 ++++ routes/api/index.js | 58 ++------------------------- routes/api/routes/message.js | 41 +++++++++++++++++++ routes/api/routes/threads.js | 23 +++++++++++ routes/api/routes/user.js | 22 ++++++++++ tests/{getmessage.py => get_msg.py} | 2 +- tests/get_thread.py | 31 ++++++++++++++ tests/{postmessage.py => post_msg.py} | 2 +- 10 files changed, 143 insertions(+), 70 deletions(-) create mode 100644 routes/api/ApiResponse.js create mode 100644 routes/api/routes/message.js create mode 100644 routes/api/routes/threads.js create mode 100644 routes/api/routes/user.js rename tests/{getmessage.py => get_msg.py} (93%) create mode 100644 tests/get_thread.py rename tests/{postmessage.py => post_msg.py} (95%) diff --git a/APIDOCS.md b/APIDOCS.md index 88e31a4..247e516 100644 --- a/APIDOCS.md +++ b/APIDOCS.md @@ -3,30 +3,27 @@ Akf-forum has got an API for other clients etc. -You can find an example in apitest.py. +You can find examples in `/tests` folder. ## Authorization You need this headers for send request to API: -```jsonc +```json { "username": "testUser", "password": "testPassword" } ``` - ## How to request? ### Request type: - `GET /api/action/id` + `GET /api/action` ### "action" types: -- `message` - -(for now, only message.) - -### "id": -ID for action type. +- GET `messages/:id` +- GET `users/:id` +- GET `threads/:id` +- POST `messages` ### Example request: diff --git a/README.md b/README.md index fd5515a..b29910c 100644 --- a/README.md +++ b/README.md @@ -34,7 +34,7 @@ And, you can learn informations about API in `APIDOCS.md`. - [ ] Singature & About me - [ ] Edit user - [ ] Messages - - [x] Ratelimit for sending message + - [ ] Ratelimit for sending message - [x] Send message - [x] Delete message - [ ] Edit message @@ -44,13 +44,17 @@ And, you can learn informations about API in `APIDOCS.md`. - [ ] Edit it! - [ ] Delete it! - [ ] Other - - [x] API - - [x] Other client for forum via API - [x] Footer of the site - [ ] Multiple theme support - [ ] Search - [x] New Thread theme, better render for messages - [ ] sending message etc. Will turn api model +- [ ] API + - [x] Other client for forum via API + - [ ] Deleting message + - [x] Sending message + - [ ] Open thread + - [x] Get Thread info ## Image: 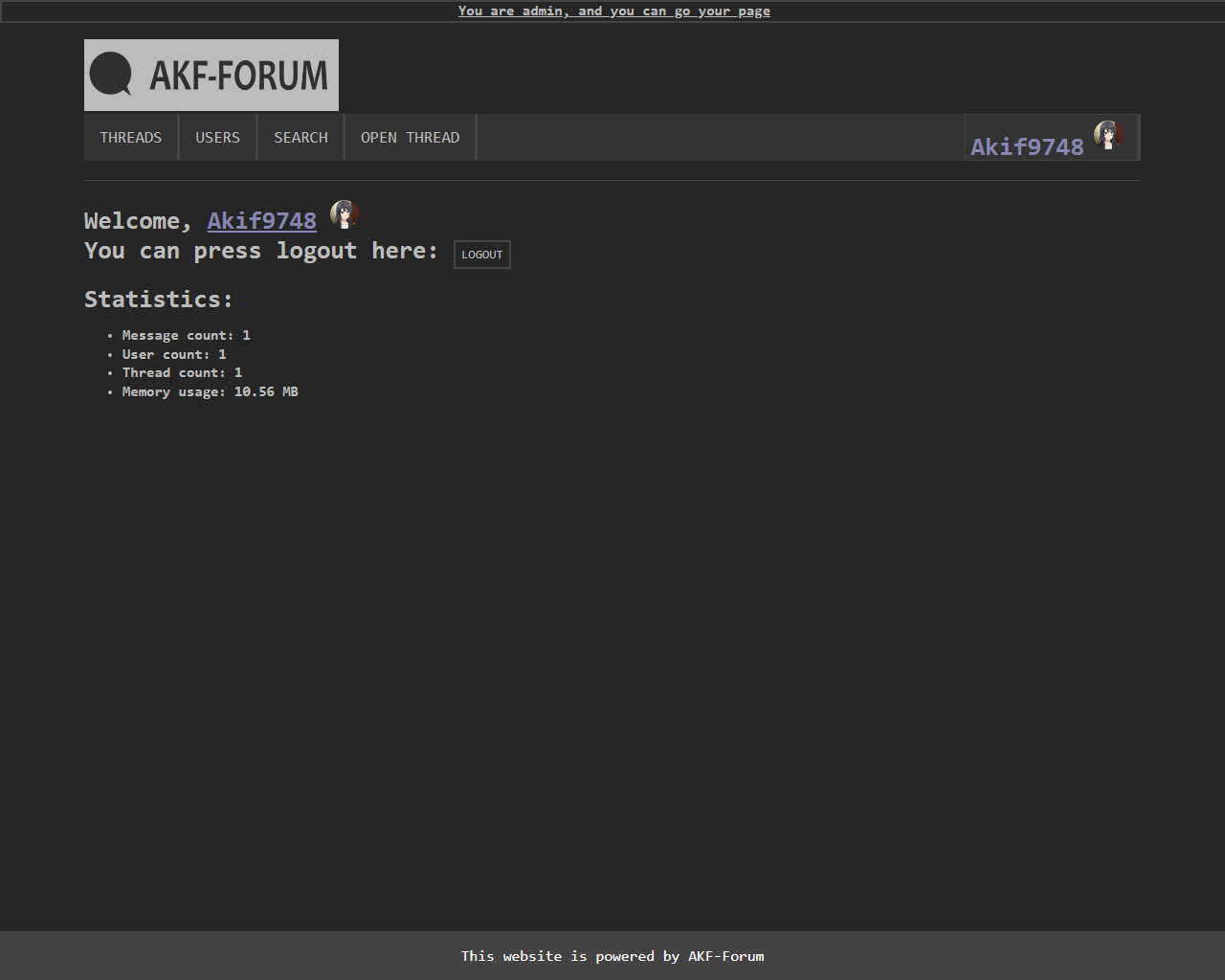 diff --git a/routes/api/ApiResponse.js b/routes/api/ApiResponse.js new file mode 100644 index 0000000..39b9280 --- /dev/null +++ b/routes/api/ApiResponse.js @@ -0,0 +1,7 @@ +class ApiResponse { + constructor(status, result) { + this.status = status; + this.result = result; + } +} +module.exports = ApiResponse; \ No newline at end of file diff --git a/routes/api/index.js b/routes/api/index.js index 7af832f..ab42145 100644 --- a/routes/api/index.js +++ b/routes/api/index.js @@ -3,12 +3,6 @@ const db = require("quick.db"); const { Router } = require("express") const app = Router(); -class ApiResponse { - constructor(status, result) { - this.status = status; - this.result = result; - } -} const { request, response } = require("express"); @@ -59,55 +53,9 @@ app.use((req, res, next) => { next(); }) -app.get("/message/:id", (req, res) => { - - const error = (status, error) => - res.status(status).json(new ApiResponse(status, { error })); - - - - const { id = null } = req.params; - if (!id) return error(400, "Missing id in query") - const message = new Message().getId(id); - - if (!message || message.deleted) return error(404, "We have not got any message declared as this id."); - - res.status(200).json(new ApiResponse(200, message)); - - - -}) - -app.post("/message/", (req, res) => { - - const error = (status, error) => - res.status(status).json(new ApiResponse(status, { error })); - const { threadID = null, content = null } = req.body; - const thread = new Thread().getId(threadID); - if (!req.body.content) return error(400, "Missing message content in request body."); - if (!thread) return error(404, "We have not got this thread."); - - - const message = new Message(content, new User().getName(req.headers.username), thread).takeId().write(); - thread.push(message.id).write(); - - res.status(200).json(new ApiResponse(200, message)); - -}) -app.get("/user/:id", (req, res) => { - - const error = (status, error) => - res.status(status).json(new ApiResponse(status, { error })) - - - const { id = null } = req.params; - if (!id) return error(400, "Missing id in query") - const member = new User().getId(id); - if (!member || member.deleted) return error(404, "We have not got any user declared as this id."); - - res.status(200).json(new ApiResponse(200, member)); - -}); +app.use("/messages", require("./routes/message")) +app.use("/users", require("./routes/user")) +app.use("/threads", require("./routes/threads")) app.all("*", (req, res) => res.status(400).json(new ApiResponse(400, { error: "Bad request" }))); diff --git a/routes/api/routes/message.js b/routes/api/routes/message.js new file mode 100644 index 0000000..0ba555d --- /dev/null +++ b/routes/api/routes/message.js @@ -0,0 +1,41 @@ +const { User, Message, Thread } = require("../../../classes"); +const ApiResponse = require("../ApiResponse"); + +const { Router } = require("express") + +const app = Router(); + +app.get("/:id", (req, res) => { + + const error = (status, error) => + res.status(status).json(new ApiResponse(status, { error })); + + const { id = null } = req.params; + if (!id) return error(400, "Missing id in query") + const message = new Message().getId(id); + + if (!message || message.deleted) return error(404, "We have not got any message declared as this id."); + + res.status(200).json(new ApiResponse(200, message)); + +}) + +app.post("/", (req, res) => { + + const error = (status, error) => + res.status(status).json(new ApiResponse(status, { error })); + const { threadID = null, content = null } = req.body; + const thread = new Thread().getId(threadID); + + if (!content) return error(400, "Missing message content in request body."); + if (!thread) return error(404, "We have not got this thread."); + + + const message = new Message(content, new User().getName(req.headers.username), thread).takeId().write(); + thread.push(message.id).write(); + + res.status(200).json(new ApiResponse(200, message)); + +}) + +module.exports = app; \ No newline at end of file diff --git a/routes/api/routes/threads.js b/routes/api/routes/threads.js new file mode 100644 index 0000000..8d6a49c --- /dev/null +++ b/routes/api/routes/threads.js @@ -0,0 +1,23 @@ +const { Thread } = require("../../../classes"); +const ApiResponse = require("../ApiResponse"); +const { Router } = require("express") + +const app = Router(); + +app.get("/:id", (req, res) => { + + const error = (status, error) => + res.status(status).json(new ApiResponse(status, { error })) + + + const { id = null } = req.params; + if (!id) return error(400, "Missing id in query") + + const thread = new Thread().getId(id); + if (!thread || thread.deleted) return error(404, "We have not got any thread declared as this id."); + + res.status(200).json(new ApiResponse(200, thread)); + +}); + +module.exports = app; \ No newline at end of file diff --git a/routes/api/routes/user.js b/routes/api/routes/user.js new file mode 100644 index 0000000..8e733f2 --- /dev/null +++ b/routes/api/routes/user.js @@ -0,0 +1,22 @@ +const { User } = require("../../../classes"); +const ApiResponse = require("../ApiResponse"); +const { Router } = require("express") + +const app = Router(); + +app.get("/:id", (req, res) => { + + const error = (status, error) => + res.status(status).json(new ApiResponse(status, { error })) + + + const { id = null } = req.params; + if (!id) return error(400, "Missing id in query") + const member = new User().getId(id); + if (!member || member.deleted) return error(404, "We have not got any user declared as this id."); + + res.status(200).json(new ApiResponse(200, member)); + +}); + +module.exports = app; \ No newline at end of file diff --git a/tests/getmessage.py b/tests/get_msg.py similarity index 93% rename from tests/getmessage.py rename to tests/get_msg.py index b94cb90..faaf4bc 100644 --- a/tests/getmessage.py +++ b/tests/get_msg.py @@ -7,7 +7,7 @@ headers = { } -r = requests.get("http://localhost:3000/api/message/1/", headers=headers) +r = requests.get("http://localhost:3000/api/messages/1/", headers=headers) print(r.json()) diff --git a/tests/get_thread.py b/tests/get_thread.py new file mode 100644 index 0000000..88c55e0 --- /dev/null +++ b/tests/get_thread.py @@ -0,0 +1,31 @@ +import requests + +# Headers for login to Akf-forum +headers = { + "username": "testUser", + "password": "testPassword" +} + + +r = requests.get("http://localhost:3000/api/threads/0", headers=headers) + +print(r.json()) + +example_response = { + "status": 200, + "result": { + "author": { + "name": "Akif9748", + "avatar": "https://www.technopat.net/sosyal/data/avatars/o/298/298223.jpg?1644694020", + "time": 1647895891332, + "admin": False, + "deleted": False, + "id": 0 + }, + "title": "First Thread", + "messages": [0, 1, 2, 3], + "time": 1647895907054, + "deleted": False, + "id": 0 + } +} diff --git a/tests/postmessage.py b/tests/post_msg.py similarity index 95% rename from tests/postmessage.py rename to tests/post_msg.py index e11c811..c59566c 100644 --- a/tests/postmessage.py +++ b/tests/post_msg.py @@ -12,7 +12,7 @@ body = { "threadID": 1 } -r = requests.post("http://localhost:3000/api/message/", +r = requests.post("http://localhost:3000/api/messages/", headers=headers, data=body) print(r.json())